Player 이동 구현
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMove : MonoBehaviour
{
public float maxSpeed;
Rigidbody2D rigid;
SpriteRenderer spriteRenderer;
Animator anim;
// Start is called before the first frame update
void Awake() {
rigid = GetComponent<Rigidbody2D>();
spriteRenderer = GetComponent<SpriteRenderer>();
anim = GetComponent<Animator>();
}
// Update is called once per frame
void Update()
{
//Stop Speed
if(Input.GetButtonUp("Horizontal")){
rigid.velocity = new Vector2(rigid.velocity.normalized.x*0.2f, rigid.velocity.y);
}
//Direction Sprite
if(Input.GetButtonDown("Horizontal")){
spriteRenderer.flipX = Input.GetAxisRaw("Horizontal") == -1;
}
//Walk <-> Breath
if(Mathf.Abs(rigid.velocity.x)<0.3f)
anim.SetBool("isWalking",false);
else
anim.SetBool("isWalking",true);
}
void FixedUpdate()
{
//Move By Control
float h=Input.GetAxisRaw("Horizontal");
rigid.AddForce(Vector2.right*h,ForceMode2D.Impulse);
if(rigid.velocity.x > maxSpeed)
rigid.velocity = new Vector2(maxSpeed, rigid.velocity.y); // Right Max Speed
else if(rigid.velocity.x < maxSpeed*(-1))
rigid.velocity = new Vector2(maxSpeed*(-1), rigid.velocity.y); // Left Max Speed
}
}
단발적인 키 입력은 Updated에서, 계속적인 키 입력은 FixedUpdate에서 해준다.
Unity에 있는 기능들을 C# 스크립트로 불러와 적절하게 사용할 수 있다. 이때, 초기화 해주는 것을 잊지 말자.
public으로 maxSpeed 변수를 생성해주면 유니티에서 값을 넣어주는 칸이 생성되어 편하다.
normalized - 벡터의 크기를 1로 만든 상태이다. (단위벡터)
선형대수학을 배울 때 단위벡터는 방향을 나타내기 위해 많이 쓰였는데 유니티에서도 마찬가지다.
python에서의 절댓값 함수 abs와 같은 기능을 하는 것은 Mathf.Abs이다.
Mathf는 수학 관련 함수를 제공하는 class이다.
Friction
player가 원활하게 움직일 수 있도록 Physics Material 2D를 생성해 마찰력을 적당히 지정해준다.
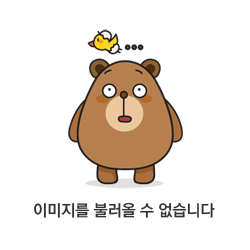
참고로 Friction이 마찰력이며, 0으로 지정해줄 경우 마찰력이 없는 빙판과 같다.
오르막길을 잘 오를 수 있도록 하기 위해서 마찰력을 0으로 설정해주었다.
만든 재질을 지형의 Collider 2D - Material에 끌어다 넣어주면 잘 적용된다.
Rigid Body에서 공기저항
Linear Drag - 공기저항, 이동 시 속도를 느리게 해준다. 보통 1~2로 설정해준다.
Animator 설정
움직이는 상태, 멈추고 있는 상태 등 상황에 따라 나타낼 player의 animation은 다르므로 코드와 함께 animator를 수정해줘야 한다. breath가 default state인 상태에서 player가 움직일 경우에는 walk 동작을 해야하므로 마우스 우클릭을 통해 breath에서 walk로의 화살표를 만들어 줘야한다. 움직이다가 멈추는 경우도 있으므로 walk에서 breath를 향하는 화살표 또한 만들어 줘야 한다.
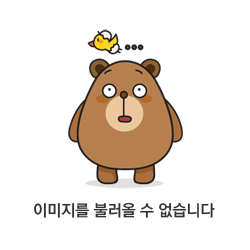
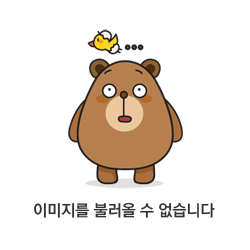
<해야 할 것>
1. Has Exit Time끄기
2. 구간닫기
3. 매개변수 설정
'game > unity(C#)' 카테고리의 다른 글
나만의 리듬게임 개발일지 - 1 (0) | 2022.07.27 |
---|---|
2D 기초 - 3 (Jump 구현) (0) | 2022.07.21 |
2D 기초 - 1 (Component, Animation) (0) | 2022.07.12 |
Visual Studio Code 자동 완성이 되지 않는 오류 (0) | 2022.07.12 |
0404) C# 기초 - 3 (0) | 2022.04.04 |